For a while now, I've been wanting to make a series of tutorials on programming your own games and game engines. I think that the main reason why most people don't want to get started with game programming, or the reason that they get put off game programming, is because there are so many pieces of information out there, and so many different things you need to learn before you can make even the simplest of games. For instance:
- You have to get a compiler or IDE (most people don't even know what these are), before you can start writing programs.
- You have to learn your programming language (such as C++) from scratch, and know it inside out. This takes time.
- You have to learn a game library or graphics API before you can start making applications (and games, eventually)
- You have to learn how to integrate all of these things in order to make a game.
Most people will do just one of these things and then give up, simply because the information is so scattered and difficult to find. What I hope to do with this tutorial series is place all of this information in one place, so that you guys can just read through these tutorials and be able to have something to show by the end! So, let's dive right in:
1. Firstly, you will have to download a compiler or IDE. Do not be put off by these complex words and phrases, a compiler is simply an application which takes the programming code that you write, and translates it into computer code (so that the computer can run your program!) For instance, you could program your entire game in notepad, but in order to actually be able to play your game, you would need to run your code through the compiler. That's it.
Probably the best compiler out there for Windows is Microsoft Visual C++. It is free, trusted and very easy to use. You can grab a copy of Microsoft Visual C++ from Microsoft.com. Just select your language (presumably English), and follow the download and install instructions.
Once you have your compiler (Visual C++) set up, you can actually begin programming!
2. You will need to start a new C++ project. Click on the 'File' menu, go to 'New' and then 'Project'.
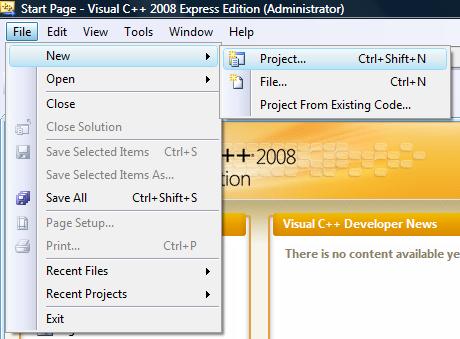
This is how you start a new Project in Visual C++
Our first applicatation with C++ will be a simple 'Hello World' application in a console window. So, click on the icon which says 'Win32 Console Application'. Type in a suitable name for the project, such as 'Hello World' in the box labelled 'Name:', and hit OK.
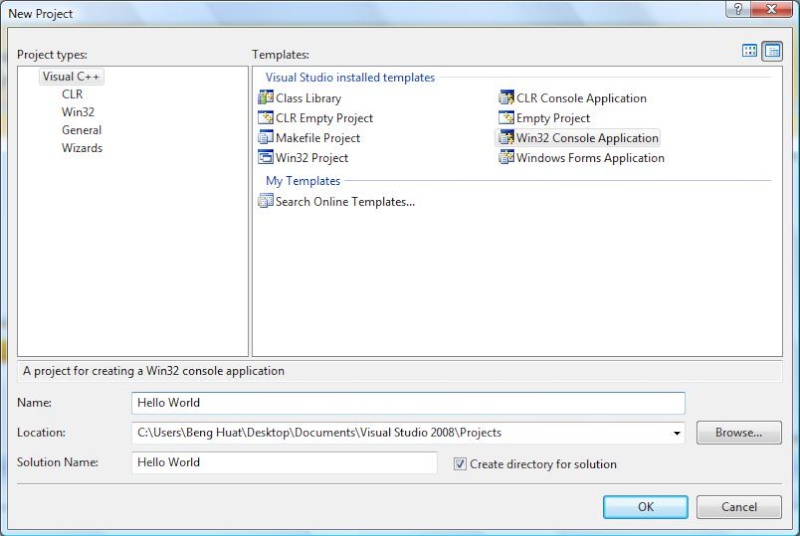
Start a new Win32 Console Application named 'Hello World'
Click 'Finish' on the Wizard that will appear when you click OK. Your project will then be generated, and Visual C++ will automatically create all of the code neccessary to start programming your console application. The code that appears will look something like this:
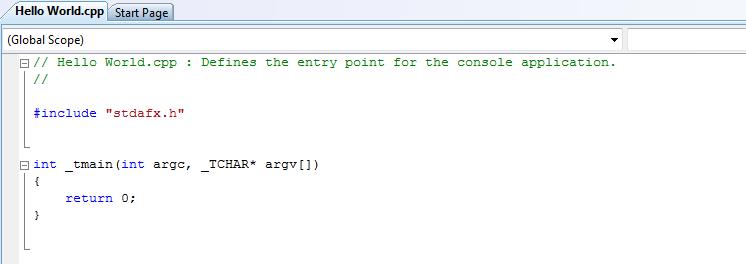
Visual C++ generates all of the boring base-code for you!
3. Now, let's start to look at exactly what this code means:
/
The first two lines of code are not really lines of code at all. They are comments, intended to make it easier to see what is actually going on. The compiler just ignores these when it runs your application; in fact, any line which starts with // is a comment, ignored by the compiler.
This line can be a little confusing. In order for us to start making a console application, the computer needs all the instructions on how to display text to the screen etc. Windows can get all of this information from the file 'stdafx.h', which contains these instructions (which saves you from having to type the instructions in yourself). You probably still don't understand why it's there, but we'll discuss that in greater detail later.
{
'_tmain' designates the main chunk of code in your program. This is where most of your program will be written, in between the '{' and the '}', which in C++ show the beginning and end of important 'chunks' of code. You do not need to understand the stuff in the brackets, that's just dumb Windows stuff. Ignore it.
This line at the end of the '_tmain' chunk tells the compiler to return to Windows regularly once the application has been successfully run. Clearly, this line of code is very important.
4. Actually, you can now run your program (which Visual C++ has generated) as it is, although it obviously won't do much as you haven't actually programmed it to do anything. However, if you press F5 to compile and run your program, then click 'Yes' when it asks if you want to build it, it will take a second to compile, and then a black console window will appear and then quickly disappear again.
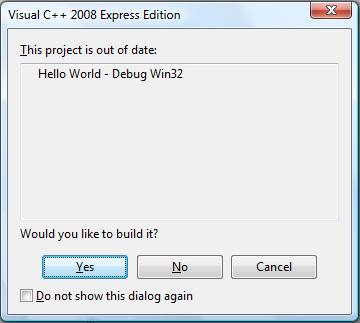
Click 'Yes' to build/compile your program.
5. So, the last real thing that we need to do is to tell Windows to keep the console window open, as well as display some text inside it. In order to do this, we will have to include the 'iostream' library in order to draw text to the screen. Add the following code after the '#include "stdafx.h"' line at the start of the program:
This includes the 'iostream' library with the project, and tells it to use the 'std' namespace, which contains most of the useful functions for input and output in Windows. Finally, add the following code inside the '_tmain' segment, before the 'return 0;' statement:
cin.get();
So, the whole program should look like this:
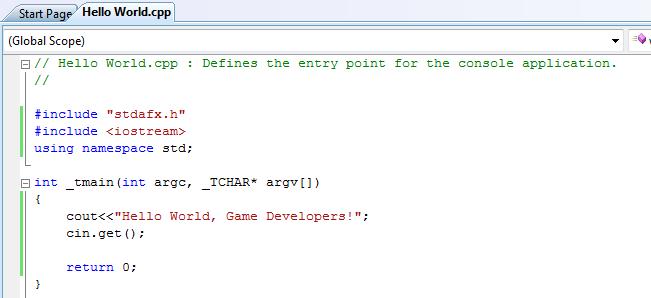
Our finished program!
6. Now you're done! Compile the program by pressing F5, click 'Yes' on the Build Window, and the console window should appear:
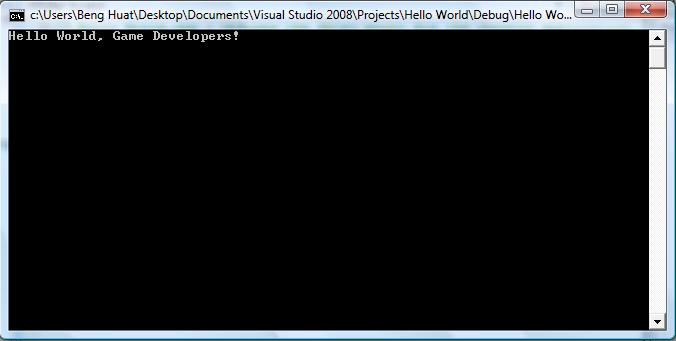
Congratulations, you've just written your first C++ program! Look out for futher tutorials, where I'll be discussing more topics on C++, until finally getting on to how you can use C++ for developing your own 2D and 3D games and engines.
See you soon!
Thankful I have always wanted to know how to program in C++ but could never find any decent tutorials but thanks to you I will be learning from your tutorials, Thankyou.
Thanks, it's good to know that people will be following our tutorial series!
Sorry about the slightly newbish tutorial on how to write a Hello World, it's just that I want to have covered some basic C++ in these tutorials before I go on to more complex concepts such as Input Systems, Graphics APIs and finally, how to make a game.
Not nooby at all! You've always got to start at the simple things!