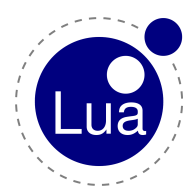
The new Lua scripting functionality offers you a lot of possibilities. There are plenty events and function available, and we're continuously extending it. You can also request any function or event if you think they're missing, simply create a topic in our forum: Lua scripting section
Here I want to show you how to set up a very simple basic script. What we want to do: We want to display the servername on the players screen when he connects, we also want to create a simple command that gives us the current time, and finally we want to prevent the player from destroying any blocks.
Let's begin!
Step 1: Set up a new script
Go to the "scripts" folder inside your game folder (if it does not exist, simply create it) and create a new folder named as your script (in our case we simply call it "BasicScript\").
Now you need the script itself (a .lua file) and a definiton.xml, which contains all required information. Find a template in our forum: Forum.concept-game.net
<Lua-Script>
<Name>BasicScript</Name>
<Description>This is a simple example script</Description>
<File>basicscript.lua</File>
<Author>red51</Author>
<Team>JIW-Games</Team>
<Website>Concept-game.net</Website>
<License>N/A</License>
</Lua-Script>
The scriptfile itself requires an event to be present, it's called "onEnable()" (which is triggered when the script is loaded).
You may print a small hint, that your script is loaded now (note: all print()-calls also occurs in the java console):
print("MyScript loaded successfully!");
end
Step 2: Accessing the server
This is quite easy. You can access the server by calling the function "getServer()". You can save it as a global variable on top of your script:
server = getServer();
function onEnable()
print("MyScript loaded successfully!");
end
Step 3: Get notified when a player spawns
To display the servername on the player screen, we need to know when he spawns, so we grab to "PlayerSpawn"-Event. We also could have used the "PlayerConnect"-Event, but since the player is still in the loadingscreen at the moment when the event is triggered, he won't see any labels on his screen.
A general note: You have to link events (with the exception of "onEnable()" and "onDisable()") with a function by calling "addEvent(eventname, function)". See:
-- some code
end
addEvent("PlayerSpawn", onPlayerSpawn);
Step 4: Display the server's name on the player's screen
To create a label, we have access to a class "Gui", which offers all required functions. To create a label, we call "Gui:createLabel(text, x, y);". Note that x and y are relative coordinates (0-1). This function returns a label object.
Now we can setup the label by changing the size or color of it.
To display the label on the player's screen, we have to call a playerfunction "player:addGuiElement(label);".
To access the player, we can grab the "event"-object provided with every call of an event. The "event"-object contains a reference to the player (this is true for all playerevents).
One more thing to do: Make the label disappear after a given amount of time, for example 5 seconds. To do this, you can create a timer. A timer requires a function it should call, the interval, the amount of repetitions, and optionally some arguments for the function. For our script, we don't need any arguments, and the function we provide should only be called 1 times by the timer.
Our "PlayerSpawn" event looks like this now:
-- create a new label
local label = Gui:createLabel("Welcome to "..server:getServerName().."!", 0.98, 0.135);
-- change the fontcolor to a nice blue
label:setFontColor(0x0066FFFF);
-- change the fontsize (default: 12)
label:setFontsize(48);
-- change the pivot of our label. 1 means "right bottom"
label:setPivot(1);
-- display the label on the player's screen
event.player:addGuiElement(label);
-- create a new timer to remove the label after 5 seconds
setTimer(function()
event.player:removeGuiElement(label);
Gui:destroyElement(label);
end, 5, 1);
end
addEvent("PlayerSpawn", onPlayerSpawn);
Step 5: Create a command to get the time
Now we need another event, the "PlayerCommand"-event. The provided command is accessible through the event-object.
The time can be grabbed from the server, and send to the player as a chat message.
See:
local command = event.command;
-- if the command contains any parameters, we need to split it
local cmd = StringUtils:explode(command, " ");
-- check the command
if cmd[1] == "/showtime" then
-- get a formatted time string from server
local currenttime = server:getGameTime();
-- send the time to the player using a colorcode (yellow)
event.player:sendTextMessage("[#FFFF00]Current time: ".. currenttime);
end
end
addEvent("PlayerCommand", onPlayerCommand);
Step 6: Prevent the player from destroying any blocks
This is also a quite easy task. All we have to do is to grab the "PlayerDestroyBlock"-event, and cancel it by calling "event:setCancel(true);". That's it! The block won't get destroyed.
event:setCancel(true);
end
addEvent("PlayerBlockDestroy", onPlayerBlockDestroy);
Results:
thanks for the tutorial, that clarifies a lot of things! :)
anyway, you need a wiki ;)
This comment is currently awaiting admin approval, join now to view.
Unfortunately this tutorial isn't up-to-date anymore, since the Lua API has been replaced by a the much more powerful Plugin API:
Forum.rising-world.net
Forum.rising-world.net
Forum.rising-world.net
Javadoc.rising-world.net